
Robert Urbańczyk
2 February 2024, 13 min read
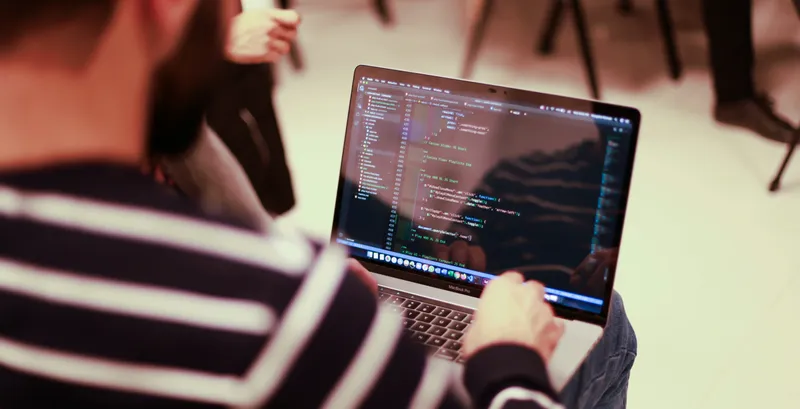
What's inside
- Why Do We Love Frameworks?
- Django or FastAPI
- Unique Selling Points
- How to Merge Them
- When to Apply
- Summary
FastAPI has been rapidly gaining popularity over the last years due to its exceptional performance, ease of use, and robust support for asynchronous programming, which are particularly advantageous for building modern, high-speed web APIs.
On the other side of the spectrum, there is Django, highly favored for full-blown web applications, offering a rich set of features like an ORM, an admin panel, and built-in security, which streamline the development of complex, database-driven web applications.
In this article, I will try to explain how to merge those two frameworks into one elegant solution.
Why Do We Love Frameworks?
Delving into the realm of software development, it's crucial to understand the concept of frameworks, foundational tools that shape and expedite the creation of robust and efficient applications.
Frameworks are loved because they are:
- Pre-designed, standardized set of tools, libraries, and practices
- Provides a structured way to build an app
- Easy to maintain
- Enhances code efficiency
- Promote best practices
- Enables faster and more robust creation of web applications
Django or FastAPI
Comparative Analysis of Django and FastAPI Features
Feature | Django | FastAPI |
---|---|---|
Python based | ✓ | ✓ |
Can deal with REST clients over HTTP | ✓ | ✓ |
Native database support | ✓ | |
Automatic documentation (OpenAPI) | ✓ | |
Validation and serialization | ✓ | ✓ |
Asynchronous support | ✓ | ✓ |
Good choice for full-stack web app | ✓ |
This table highlights the key differences and strengths of each framework. Note that while both have capabilities for validation, serialization, and asynchronous support, these are more inherent and central features in FastAPI. Django's traditional strength lies in its suitability for full-stack web applications.
Unique Selling Points
In web development, choosing the right framework can be pivotal to the success of a project. With this in mind, it's important to explore the unique selling points of both frameworks:
Django
- Extensive and well-maintained documentation. Django offers comprehensive and regularly updated documentation, making it easier for developers to learn and use the framework effectively.
- “Batteries included” philosophy. This framework comes with numerous built-in features for common web development tasks, reducing the need for additional plugins or modules.
- ORM and DB abstraction. Django's Object-Relational Mapping system simplifies database operations by allowing developers to use Python code instead of SQL.
- Low-code admin interface. It provides a ready-to-use admin interface that lets you manage data with minimal coding, enhancing productivity.
- Huge community. A large and active community means better support, abundant resources, and frequent updates.
- Security features (XSS, CSRF, SQL injection). Django includes built-in protections against many common security threats, offering a safer development environment.
- Versatility and modularity. The framework's modular nature allows for flexible application development and customization.
FastAPI
- Fast and reliable REST API framework. Optimized for speed and performance, FastAPI is ideal for building high-performance RESTful APIs.
- Easy to use, low entry point. Its straightforward and intuitive design makes FastAPI accessible to beginners while being powerful enough for experienced developers.
- Flatter learning curve. Developers can quickly become proficient in FastAPI due to its simplicity and clear documentation.
- Dependency injection system. This feature simplifies the management of dependencies, leading to cleaner and more maintainable code.
- Type hints for validation. FastAPI uses Python type hints to validate data, reducing bugs and improving the clarity of the code.
- Auto documentation with OpenAPI standard. The framework automatically generates interactive documentation using the OpenAPI standard, making API testing and exploration easier.
- Performance. FastAPI's performance is comparable to NodeJS and Go, thanks to its Starlette and Pydantic foundations, offering high-speed data processing and API responses.
If you are looking for a detailed comparison between these two frameworks check our blog post written by our Senior Python Developer Patryk Młynarek - Django vs. FastAPI
How to Merge Them
In this section, I will present four distinct scenarios illustrating how Django and FastAPI can be integrated practically, each time highlighting the advantages and drawbacks of the proposed solution.
Scenario A: Separate Codebases
Scenario A involves maintaining separate codebases for logic, with the API and web UI interface hosted on different domains.
Scenario A: Separate Codebases for API and Web UI on Different Domains.
Pros of Scenario A:
- Specialization and Focus: By keeping the API and web UI logic separate, each can be developed and optimized for its specific use case, leading to more efficient and focused code.
- Scalability: This approach allows for independent scaling of the web interface and the API, which is beneficial if one component experiences more traffic or requires more resources than the other.
- Security: Hosting on different domains can enhance security. It separates concerns and reduces the risk of one part of the system compromising the other.
- Flexibility in Development and Deployment: Developers can work on the API and the web UI independently, potentially using different technologies or libraries suited to each part. This also allows for separate deployment schedules.
- Ease of Maintenance: Issues in one system (API or web UI) are less likely to directly impact the other, simplifying maintenance and troubleshooting.
Cons of Scenario A:
- **Complexity in Integration: Separate codebases can lead to challenges in maintaining consistency and ensuring seamless integration between the API and the web UI.
- Increased Overhead: Managing two separate systems can result in increased operational complexity, requiring more effort in terms of deployment, monitoring, and ensuring both systems work harmoniously.
- Cross-Domain Communication Issues: Having the API and web UI on different domains might introduce complexities with cross-domain requests, potentially leading to issues with CORS (Cross-Origin Resource Sharing).
- Duplication of Effort: Some logic might need to be implemented in both codebases, leading to duplication of effort and potential inconsistencies.
- Potential Performance Impact: Depending on the architecture, having separate domains can introduce latency in communication between the API and the web interface, potentially impacting user experience.
Scenario B: Two Separate Domains
In Scenario B we still have two separate domains, but this time with FastAPI using Django's business logic layer. This setup leverages the strengths of both frameworks but requires careful planning and execution to effectively integrate and manage them.
This integration facilitates the use of Django ORM's sophisticated and feature-rich models and queries within a FastAPI setting by enabling the reuse of Django project codebase.
Scenario B: Two Separate Domains with FastAPI Using Django's Business Logic.
Pros of Scenario B:
- Leveraging Django’s Robustness: By utilizing Django’s business logic, you benefit from its mature and well-tested framework for handling complex business operations.
- Performance Boost: FastAPI's performance capabilities are used for handling API requests, which can significantly improve response times and efficiency, especially for asynchronous tasks.
- Best of Both Worlds: This approach allows you to combine the robust features and security of Django with the speed and modern architecture of FastAPI.
- Independent Scalability: Each domain (the Django web application and the FastAPI service) can still be scaled independently according to their specific load and performance requirements.
- Simplified Maintenance for Business Logic: Since the business logic resides in Django, any updates or changes need to be made in one place, ensuring consistency.
Cons of Scenario B:
- Complex Integration: Ensuring that FastAPI correctly and efficiently interacts with Django's business logic can add a layer of complexity and might require custom solutions.
- Increased Development Overhead: Developers need to be proficient in both Django and FastAPI, and there might be extra work in making sure that both systems communicate effectively.
- Dependency on Django’s Structure: FastAPI’s architecture might be constrained by Django’s business logic design, potentially limiting some of FastAPI’s flexibility and speed advantages.
- Cross-Domain Complications: Similar to Scenario A, managing cross-domain requests can introduce challenges, particularly in terms of security (like CORS issues) and data synchronization.
Scenario C: Single Domain
In Scenario C, we bundle together Django and FastAPI under a single domain. This scenario seeks to merge the strengths of both FastAPI and Django, while maintaining separate processes for efficiency and specialization.
Scenario C: Single Domain with Separate Processes, FastAPI Utilizes Django Business Logic for REST API Requests.
Pros of Scenario C:
- Unified Domain Structure: Utilizing a single domain streamlines both the setup and deployment processes, particularly for web applications where the API is consumed directly by the web app itself.
- Performance Optimization: FastAPI handles REST API requests in a separate process, leading to more efficient handling of API calls, especially for asynchronous operations.
- Consolidated Business Logic: By using Django's business logic, there is a central place for the business rules and data handling, ensuring consistency across different parts of the application.
- Flexibility in Using FastAPI’s Features: FastAPI's architecture can be fully leveraged for API requests, providing benefits like faster request handling.
- Reduced Cross-Domain Issues: Eliminates the complications associated with cross-domain requests, such as CORS, as both the API and web interface are served under the same domain.
Cons of Scenario C:
- Complex System Architecture: Managing two separate processes under a single domain can be complex, requiring careful configuration and monitoring to ensure seamless operation.
- Resource Contention: If not managed well, both processes could compete for system resources, leading to performance bottlenecks.
- Integration Challenges: Ensuring effective communication and integration between FastAPI and Django’s business logic can be intricate and may require custom development work.
- Dependency on Django’s Design: FastAPI's capabilities may be limited or influenced by the underlying Django architecture, which could restrict its full potential.
- Troubleshooting Complexity: With two separate processes under the hood, diagnosing and fixing issues can be more challenging, as it requires understanding and navigating both FastAPI and Django systems.
Scenario D: Run Both Frameworks in One Process
In this scenario, FastAPI and Django are integrated closely, offering a streamlined setup with a single process. This approach is advantageous for simpler deployment and management, but it requires careful configuration and monitoring.
Scenario D: FastAPI and Django Running in One ASGI Process, FastAPI Encapsulates Django Using application.mount
Pros of Scenario D:
- Integrated System: Running both FastAPI and Django within a single ASGI process simplifies deployment and application management, as they share the same runtime environment.
- Seamless Routing: FastAPI can handle API requests directly, while non-API requests (anything other than /api) are seamlessly routed to Django, offering a clean separation of concerns within the same process.
- Optimized Performance: With both frameworks running in a unified process, the overhead associated with inter-process communication is eliminated, potentially improving performance.
- Simplified Architecture: This setup reduces the complexity compared to managing multiple domains or processes, making the system easier to understand and maintain.
- Efficient Resource Utilization: Sharing the same process can lead to more efficient use of server resources, as both frameworks can leverage the same memory and CPU resources.
Cons of Scenario D:
- Complex Configuration: Setting up FastAPI to encapsulate Django and route requests appropriately can be complex and may require in-depth knowledge of both frameworks.
- Tight Coupling: Since both frameworks run in the same process, changes or issues in one framework could directly impact the other, leading to a tightly coupled system.
- Scalability Considerations: Scaling a single process that runs both frameworks might be more challenging compared to scaling them independently, especially for applications with high traffic.
- Risk of Resource Contention: Both frameworks sharing the same process can lead to contention for resources like memory and CPU, which might affect performance under heavy load.
- Dependency and Compatibility Issues: Keeping both FastAPI and Django updated and ensuring compatibility between them within the same environment could present ongoing challenges.
When to Apply
Let's compare Scenarios A, B, C, and D in the context of typical use cases:
Simple CRUD-like Applications, No Logic in Django, Separated API:
- Scenario A is ideal here. With simple CRUD operations and no complex logic in Django, keeping the API separate simplifies the architecture. This scenario suits applications where you need a straightforward, decoupled API on a separate domain.
Large, Legacy Codebase in Django:
- Scenario A, B and C could be a good fit. Here, FastAPI can leverage the existing Django business logic, making it suitable for modernizing or extending a large, established Django codebase with a high-performance API layer.
Logic in Django Models, Signals, etc.:
- Scenario B, C and D are suitable. Having a deployment with separate processes allows you to utilize Django's models and signal logic while FastAPI handles the API requests.
REST API Only + Django Models and Admin Goodies (React App):
- Scenario D is the best choice for this requirement. It allows for a unified process where FastAPI encapsulates Django, making it perfect for applications where Django’s backend capabilities (like models and admin features) are needed alongside a REST API, especially in cases where a frontend framework like React is used.
A | B | C | D | |
---|---|---|---|---|
Simple CRUD-like applications, no logic in Django, separated API | ✓ | |||
Large, legacy codebase in Django | ✓ | ✓ | ✓ | |
Logic in Django models, signals, etc. | ✓ | ✓ | ✓ | |
REST API only + Django models and admin goodies (react app) | ✓ |
Integrating a large, legacy codebase often involves complex challenges in extension, refactoring and maintenance, making the addition of FastAPI as a side service a viable and strategic choice to modernize the application without disrupting the existing system.
As you can see, each scenario offers distinct advantages depending on the complexity of the application, the existing codebase, and the specific requirements of the API and web interface. The choice should align with the need for performance, simplicity, and the extent of integration between Django and FastAPI.
Summary
In this detailed exploration, I've examined how FastAPI and Django, each with their unique strengths, can be synergistically combined to create powerful, efficient web applications. From the simplicity and performance of FastAPI to the robustness and feature-rich environment of Django, understanding how to leverage these frameworks together opens up new avenues for developing cutting-edge web solutions.
Whether you're handling simple CRUD applications, managing large legacy codebases, or seeking to integrate complex business logic with high-performance APIs, the right combination of Django and FastAPI can significantly enhance your web development capabilities.
Let’s discuss how we can transform your web development strategy. Contact Sunscrapers through the form for a consultation, and let’s build something remarkable together!