
Patryk Młynarek
15 February 2024, 6 min read
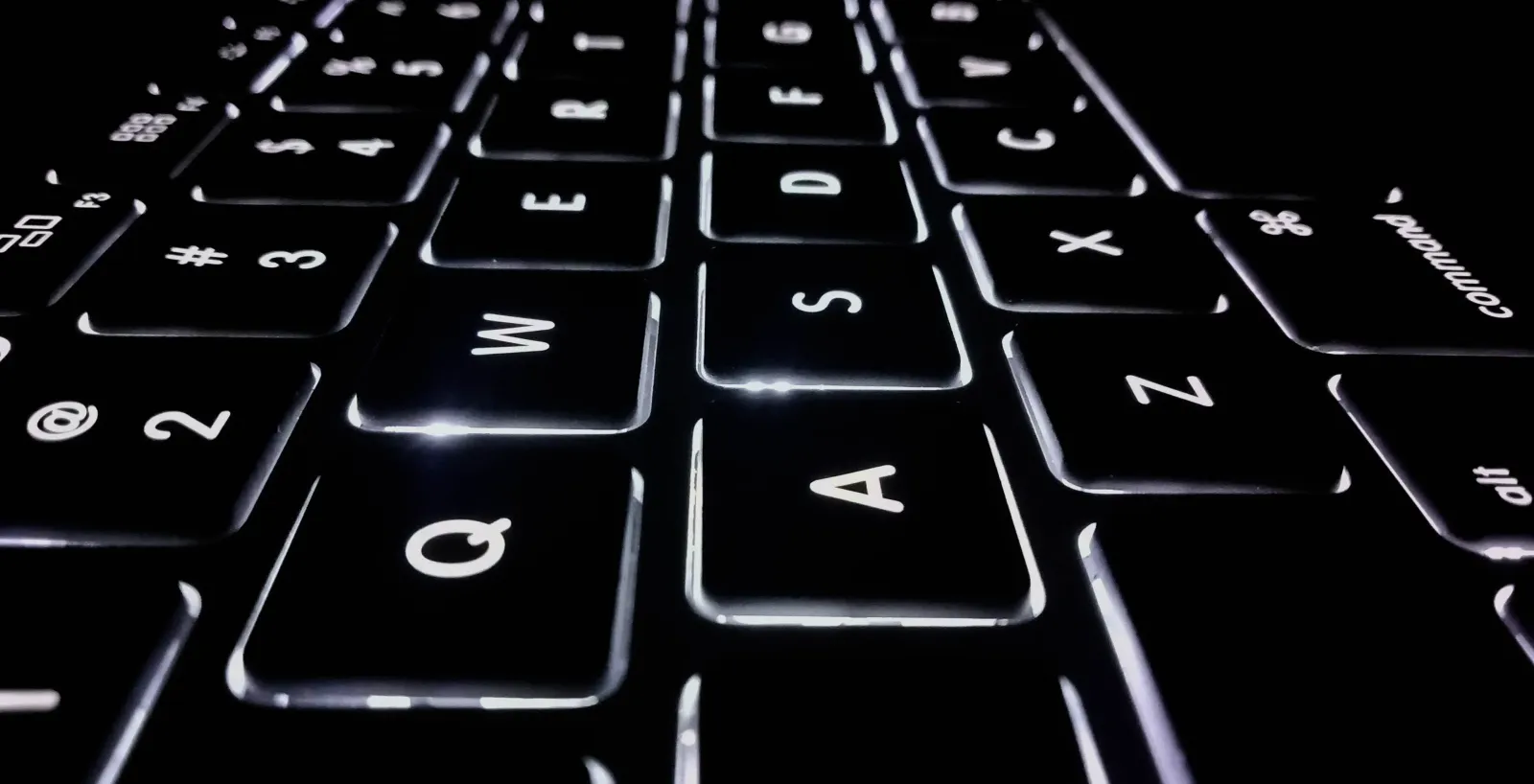
What's inside
- Django Overview
- FastAPI Insight
- Main Benefits of Django
- Main Benefits of FastAPI
- Where Django Lags
- FastAPI's Shortcomings
- Final remarks
The previous article analyzed the differences between DRF (Django Rest Framework) and FastAPI. This time, we turn our lens towards a broader perspective by contrasting FastAPI with Django.
If you missed our deep dive into DRF and FastAPI, check it out here. While some themes might seem familiar, especially given DRF's foundation on Django, distinct differences warrant exploration.
For those navigating the Python ecosystem, our seasoned team at Sunscrapers is here to provide insight and guidance cultivated from years of expertise.
Django Overview
For many, Django is a household name. But, in the essence of thoroughness, here's a snapshot:
Django is a stable and battle-tested web framework coded in Python. Abiding by the model-template-view (MTV) architecture and the DRY (Don't Repeat Yourself) principle, Django prides itself on its open-source nature. Since its inception in 2005, the framework has seen diligent maintenance through independent non-profit organizations. Notably, its latest releases also incorporate asynchronous support, showcasing the framework's commitment to staying at the forefront of modern web development.
# A basic Django view
from django.http import HttpResponse
def hello(request):
return HttpResponse("Hello, Django!")
FastAPI Insight
FastAPI, while newer, is carving a niche for itself:
FastAPI is a modern, fast (high-performance) micro-framework for building web applications with Python, especially suited for crafting APIs. It utilizes Python-type hints in tandem with Pydantic for data validation and serialization. The asynchronous capabilities and ASGI servers like Uvicorn and Gunicorn offer robust production solutions.
# A basic FastAPI endpoint
from fastapi import FastAPI
app = FastAPI()
@app.get("/hello")
async def read_root():
return {"Hello": "FastAPI"}
Main Benefits of Django
Django's strengths are rooted in its comprehensive approach, providing a robust foundation for various applications:
- Security: Django boasts a robust security framework, guarding against CSRF, SQL injections, and more. This safety net is particularly invaluable for newcomers seeking a secure development environment.
- Authentification: Django's built-in authentication system, including user models, views, and forms, is top-notch.
- Scalability & Versatility: Django can evolve with growing needs, accommodating small and large projects.
- Community: With over a decade of active presence, Django's thriving community provides a rich array of resources and an extensive collection of packages, ensuring solutions for various functionalities are readily available.
- MVT Model: Django’s distinct approach separates concerns, aiding clarity.
- Predefined and Generic Classes: Django facilitates rapid development through predefined and generic classes, reducing repetitive coding tasks and promoting code efficiency.
- Easy Debugging: Django simplifies the debugging process, providing tools and features that aid developers in identifying and resolving issues swiftly.
- Built-in Testing Tools: Django comes equipped with built-in testing tools, streamlining the testing process and ensuring the reliability and stability of applications.
from django.test import TestCase
from myapp.models import Animal
class AnimalTestCase(TestCase):
def setUp(self):
Animal.objects.create(name="lion", sound="roar")
Animal.objects.create(name="cat", sound="meow")
def test_animals_can_speak(self):
"""Animals that can speak are correctly identified"""
lion = Animal.objects.get(name="lion")
cat = Animal.objects.get(name="cat")
self.assertEqual(lion.speak(), 'The lion says "roar"')
self.assertEqual(cat.speak(), 'The cat says "meow"')
- Django ORM Efficiency: The Object-Relational Mapping (ORM) in Django is exceptionally helpful and efficient. It streamlines database management, making it easier to interact with databases, perform queries, and manage data seamlessly.
# Django Model and ORM example
from django.db import models
class User(models.Model):
username = models.CharField(max_length=100)
email = models.EmailField()
User.objects.filter(username__icontains="user").all()
- Project Maintenance: Django ORM not only simplifies database tasks but also contributes significantly to project maintenance. Its well-designed structure and functionalities enhance overall project maintainability, ensuring a smooth and hassle-free development experience.
# Some of the built in commands
dumpdata
makemigrations
migrate
runserver
startapp
startproject
shell
test
- Web Forms: ModelForms in Django seamlessly handle forms, integrating validations and protections.
from django.forms import ModelForm
from myapp.models import Article
# Create the form class.
class ArticleForm(ModelForm):
class Meta:
model = Article
fields = ["pub_date", "headline", "content", "reporter"]
# Creating a form to add an article.
form = ArticleForm()
# Creating a form to change an existing article.
article = Article.objects.get(pk=1)
form = ArticleForm(instance=article)
Main Benefits of FastAPI
FastAPI, while compact, is not lightweight:
- Performance: Benchmarks often illustrate FastAPI's edge in speed, with Starlette and Uvicorn being the only major contenders.
- Concurrency: With Python's async capabilities, FastAPI promotes efficient concurrency without the fuss:
# Async endpoint in FastAPI
@app.get('/')
async def read_results():
results = await some_library()
return results
- Automatic Documentation: Swagger and ReDoc interfaces make API interaction intuitive for developers and collaborators.
- Dependency injection & Validation: FastAPI streamlines dependencies, enhancing modularity, and employs Pydantic for robust data validations.
from typing import Annotated, Union
from fastapi import Depends, FastAPI
app = FastAPI()
async def common_parameters(
q: Union[str, None] = None, skip: int = 0, limit: int = 100
):
return {"q": q, "skip": skip, "limit": limit}
@app.get("/items/")
async def read_items(commons: Annotated[dict, Depends(common_parameters)]):
return commons
@app.get("/users/")
async def read_users(commons: Annotated[dict, Depends(common_parameters)]):
return commons
- Easy Testing: Testing FastAPI endpoints is straightforward and conducive to Test Driven Development (TDD) with the help of the TestClient provided by FastAPI. This ease of testing contributes to the development of reliable and maintainable applications.
from fastapi import FastAPI
from fastapi.testclient import TestClient
app = FastAPI()
@app.get("/")
async def read_main():
return {"msg": "Hello World"}
client = TestClient(app)
def test_read_main():
response = client.get("/")
assert response.status_code == 200
assert response.json() == {"msg": "Hello World"}
- Easy Deployment: FastAPI facilitates easy deployment, offering Docker support through its provided Docker image. Additionally, deploying to AWS Lambda is straightforward, providing versatile deployment options for developers.
- Good Community: FastAPI benefits from a vibrant and supportive community. The active engagement and contributions from the community enhance the framework's ecosystem, providing valuable resources and support for developers.
Where Django Lags
While Django is powerful, certain aspects could be refined:
- NoSQL Databases: Django's ORM predominantly caters to SQL databases. While NoSQL integrations exist, they can sometimes feel less natural.
- REST support: Out-of-the-box Django lacks RESTful capabilities, necessitating the DRF addition for robust API solutions.
- Performance: In direct speed comparisons, micro-frameworks like FastAPI often edge out.
- Learning curve Again, because of its size - the learning curve is much steeper than the one of FastAPI.
- Interactive Documentation: Django’s documentation, though vast, lacks the interactivity seen in FastAPI.
FastAPI's Shortcomings
No framework is without challenges:
- Limited Built-In Security: While FastAPI offers fastapi.security utilities, a cohesive, built-in system like Django's is absent.
- Limited Built-In Functionalities: Being a micro web framework, FastAPI intentionally keeps its core functionalities minimal. While this promotes flexibility, it means that developers may need to implement many features from scratch or find external libraries for certain functionalities.
- Learning Curve for Asynchronous Programming: FastAPI heavily utilizes asynchronous programming, which may pose a learning curve for developers unfamiliar with this paradigm.
Final remarks
So which should you choose?
Django and FastAPI serve different niches. If your project leans towards API-centric tasks, FastAPI shines. Django stands out for comprehensive applications, especially with DRF for APIs.
Of course, the final choice is always challenging. Hence, we strongly recommend consulting professionals like us so that we can make a detailed analysis based on your business needs and requirements to see which option would be better.