
Gabriel Knez
23 October 2023, 8 min read
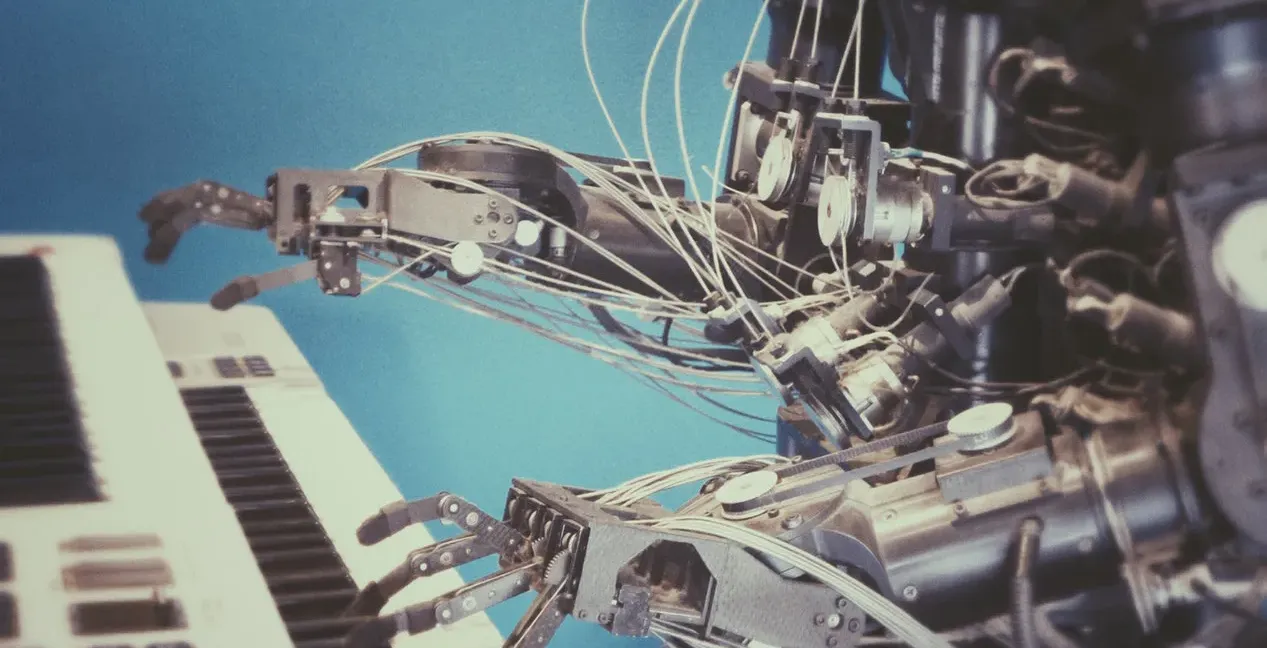
What's inside
- Top 25 Libraries You Need to Know
- Data Processing
- Machine Learning & Deep Learning
- Data Exploration
- Data Scraping
- Multi-Function
- Conclusion
- Take Action: Stay Updated and Engage with Us
Python is a go-to language for data scientists and web developers, mainly due to its extensive array of libraries that cover virtually any task, including machine learning.
If you're embarking on a data science venture that leverages machine learning, Python offers a wealth of libraries tailored to various use cases, skill levels, and customization needs.
Crafting machine learning algorithms from scratch is complex, but thankfully, the Python community has put in the legwork, creating libraries that simplify the process and save valuable development time.
Top 25 Libraries You Need to Know
Now that you understand Python's importance and versatility in the data science and machine learning landscapes, it's time to dig deeper.
But with the vast number of libraries available, where do you start? Fear not because I've done the heavy lifting for you.
Whether you're a novice dipping your toes into the machine learning pool or a seasoned data scientist searching for that perfect tool to optimize your workflow, I have something for everyone.
Below, I’ll walk you through 24 of Python's most powerful machine-learning libraries, categorized by their core functionalities and applications.
Let's dive in!
Note: This article was last updated on October 12, 2023.
Data Processing
1. NumPy
Website: https://github.com/numpy/numpy
GitHub stars: 24,7k
Contributors: 1530
Description: NumPy, short for Numerical Python, offers robust features for operations on n-arrays and matrices. This library enhances the performance of mathematical operations through array vectorization.
Applications: Primarily used in scientific computing.
Code Sample:
import numpy as np
a = np.array([1, 2, 3])
print(a + a)
2. SciPy
Website: https://scipy.org/
GitHub stars: 11,8k
Contributors: 1379
Description: SciPy builds upon NumPy and includes modules for linear algebra, integration, optimization, and statistics. It's appreciated for its well-documented, efficient numerical routines.
Applications: Scientific programming in mathematics, science, and engineering.
Code Sample:
from scipy import integrate
result, error = integrate.quad(lambda x: x**2, 0, 1)
3. Pandas
Website: https://pandas.pydata.org/
GitHub stars: 39,9k
Contributors: 3036
Description: Pandas handle labeled and relational data through two main data structures: Series and DataFrames. It offers functionalities for easy data manipulation and visualization.
Applications: Data wrangling and manipulation.
Code Sample:
import pandas as pd
df = pd.DataFrame({'A': [1, 2], 'B': [3, 4]})
4. Polars
Website: https://www.pola.rs/
GitHub stars: 20,9k
Contributors: 287
Description: Polars is a high-performance DataFrame library optimized for large data sets. It utilizes lazy evaluation and multi-threading for rapid data operations.
Applications: Data manipulation and large dataset processing.
Code Sample:
import polars as pl
df = pl.DataFrame({
"name": ["John", "Jane"],
"age": [28, 34]
})
filtered_df = df.filter(df["age"] > 30)
5. Matplotlib
Website: https://matplotlib.org/stable/
GitHub stars: 18,2k
Contributors: 1336
Description: Matplotlib is designed for generating simple yet powerful visualizations. It offers lower-level control, meaning more code may be required for complex visualizations.
Check out this step-by-step guide to data visualization in Python.
Applications: Two-dimensional plotting.
Code Sample:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3], [1, 4, 9])
plt.show()
6. Seaborn
Website: https://seaborn.pydata.org/
GitHub stars: 11,2k
Contributors: 187
Description: Seaborn, built on top of Matplotlib, focuses on the visualization of statistical models, offering a variety of complex plots.
Applications: Data visualization.
Code Sample:
import seaborn as sns
sns.set_theme()
tips = sns.load_dataset("tips")
sns.relplot(x="total_bill", y="tip", data=tips)
7. Bokeh
Website: https://bokeh.org/
GitHub stars: 18k
Contributors: 587
Description: Bokeh specializes in interactive visualizations independent of Matplotlib. It works in modern browsers and offers various interactive features.
Applications: Interactive web visualizations.
Code Sample:
from bokeh.plotting import figure, output_file, show
output_file("line.html")
p = figure()
p.line([1, 2, 3, 4, 5], [6, 7, 2, 4, 7])
show(p)
8. Plotly
Website: https://plotly.com/python/
GitHub stars: 14,2k
Contributors: 223
Description: Plotly is a web-based tool that supports many impressive visualizations. It is particularly suited for interactive web applications.
Applications: Web-based plotting.
Code Sample:
import plotly.express as px
fig = px.scatter(x=[0, 1, 2, 3, 4], y=[0, 1, 4, 9, 16])
fig.show()
9. pydot
Website: https://pypi.org/project/pydot/
GitHub stars: 806
Contributors: 21
Description: Pydot is an interface to Graphviz and is ideal for generating complex graphs.
Applications: Graph-based algorithms and structures.
Code Sample:
import pydot
graph = pydot.Dot(graph_type='graph')
edge = pydot.Edge("A", "B")
graph.add_edge(edge)
Machine Learning & Deep Learning
10. PyTorch
Website: https://pytorch.org/
Github stars: 71,2k
Contributors: 2951
Description: A successor to Torch, PyTorch offers a platform for tensor computation and dynamic computational graphs. It simplifies complex math and automates gradient calculations.
Applications: Data scientists who desire a more dynamic and hands-on approach to deep learning.
Code Sample:
import torch
x = torch.Tensor([1, 2, 3])
11. SciKit-Learn
Website: https://scikit-learn.org/
GitHub stars: 56k
Contributors: 2737
Description: SciKit-Learn is a go-to library for standard machine learning algorithms built on top of SciPy.
Applications: Various machine learning tasks, including clustering, regression, and classification.
Code Sample:
from sklearn.linear_model import LinearRegression
model = LinearRegression()
12. XGBoost
Website: https://xgboost.readthedocs.io/en/stable/
GitHub stars: 24,6k
Contributors: 603
Description: XGBoost is an optimized distributed gradient boosting library known for its efficiency and flexibility.
Applications: Gradient boosting framework.
Code Sample:
import xgboost as xgb
dtrain = xgb.DMatrix('train.txt')
params = {'objective': 'binary:logistic'}
bst = xgb.train(params, dtrain)
13. LightGBM
Website: https://lightgbm.readthedocs.io/en/latest/
GitHub stars: 15,5k
Contributors: 291
Description: LightGBM offers gradient boosting with decision tree-based algorithms and is known for its speed and efficiency.
Applications: Ranking, classification, and more.
Code Sample:
import lightgbm as lgb
train_data = lgb.Dataset('train.txt')
params = {'objective': 'binary'}
bst = lgb.train(params, train_data)
14. CatBoost
Website: https://catboost.ai/
GitHub stars: 7,4k
Contributors: 340
Description: CatBoost is a gradient-boosting library known for its speed and quality. It supports numerical and categorical data.
Applications: Ranking, classification, regression.
Code Sample:
from catboost import CatBoostClassifier
model = CatBoostClassifier()
15. Eli5
Website: https://eli5.readthedocs.io/en/latest/
GitHub stars: 2,7k
Contributors: 15
Description: Eli5 helps debug machine learning models by offering visualization tools.
Applications: Debugging machine learning models.
Code Sample:
import eli5
eli5.show_weights(model)
16. PyBrain
Website: n/a
GitHub stars: 2,8k
Contributors: 33
Description: Although inactive, PyBrain offers a range of machine-learning algorithms. It was designed for both beginners and advanced users.
Applications: General-purpose machine learning.
Code Sample: The library is inactive. The example is not applicable.
17. Keras
Website: https://keras.io/
GitHub stars: 59,4k
Contributors: 1167
Description: Keras is an open-source neural network library offering high-level, easy-to-use APIs.
Applications: Neural network modeling.
Code Sample:
from keras.models import Sequential
model = Sequential()
18. Dist-Keras
Website: https://joerihermans.com/work/distributed-keras/
GitHub stars: 624
Contributors: 4
Description: Built on Keras and Apache Spark, Dist-Keras focuses on distributed deep learning.
Applications: Distributed deep learning.
Code Sample: The library has limited examples and support.
19. Theano
Website: https://pytensor.readthedocs.io/en/latest/
GitHub stars: 163
Contributors: 413
Description: Theano was a Python library that allows you to define, optimize, and evaluate mathematical expressions involving multi-dimensional arrays efficiently.
PyTensor is a fork of Aesara - a Python library for defining, optimizing, and efficiently evaluating mathematical expressions involving multi-dimensional arrays.
Applications: Scientific computing, Data analytics, and Machine learning models.
Code Sample:
import theano
import theano.tensor as T
x = T.dscalar('x')
y = x ** 2
20. TensorFlow
Website: https://www.tensorflow.org/
GitHub stars: 178k
Contributors: 3437
Description: Born out of Google Brain, TensorFlow is a leading framework for deep and machine learning tasks. Known for its multi-dimensional arrays called tensors, it allows efficient deployment of computation across various platforms.
Applications: Deep learning enthusiasts and professionals, especially those involved in large-scale projects like object identification and speech recognition.
Code Sample:
import tensorflow as tf
x = tf.constant([1, 2, 3])
21. Caffe
Website: http://caffe.berkeleyvision.org/
Github stars: 33,6k
Contributors: 258
Description: Developed by BVLC and BAIR, Caffe specializes in vision-based machine learning tasks. It excels in image classification and convolutional neural networks.
Applications: Those focusing on machine vision applications, particularly in an academic research setting.
Code Sample: The library is often used through command lines or configuration files.
22. Fuel
Website: https://fuel.readthedocs.io/en/latest/
Github stars: 859
Contributors: 34
Description: Fuel acts as a data pipeline for machine learning models, offering out-of-the-box support for popular datasets and on-the-fly data preprocessors.
Applications: Data scientists who need to easily manage and preprocess training data.
Code Sample: The library has limited examples and support.
Data Exploration
23. StatsModels
Website: https://www.statsmodels.org/devel/
Github stars: 8,9k
Contributors: 357
Description: Ideal for statistical analysis, StatsModels provides methods for various statistical models from simple linear regression to time-series analysis.
Applications: Analysts and researchers focused on comprehensive statistical analysis and data exploration.
Code Sample:
import statsmodels.api as sm
y = [1, 2, 3]
X = [1, 2, 3]
X = sm.add_constant(X)
model = sm.OLS(y, X).fit()
Data Scraping
24. Scrapy
Website: https://scrapy.org/
Github stars: 48,6k
Contributors: 519
Description: Scrapy is a robust framework for scraping structured data from the web, supporting large-scale data extraction.
Applications: Data engineers and analysts need large volumes of web data.
Code Sample: The library is often used through the command line and separate files for spiders.
Multi-Function
25. Pattern
Website: https://github.com/clips/pattern/wiki
Github stars: 8,6k
Contributors: 19
Description: Pattern is an all-in-one solution offering machine learning algorithms, data collection, and analysis. It supports data mining from sources like Google, Twitter, and Wikipedia.
Applications: Those looking for an all-in-one solution, especially in natural language processing and data collection.
Code Sample: The Library has limited examples and support.
Read this too: 8 best Python Natural Language Processing (NLP) libraries.
Note: This article was last updated on October 12, 2023.
Note: These code snippets are simplified examples intended to illustrate the core functionalities of each library. For comprehensive documentation, it's always a good idea to check the official website of each library. For comprehensive documentation, it's always a good idea to check the official website of each library.
Conclusion
We've just scratched the surface of the world of Python machine-learning libraries. Though we've covered some incredibly versatile and powerful tools, countless others are waiting to be explored.
These libraries are not just useful but indispensable for data scientists, machine learning enthusiasts, and software engineers serious about building cutting-edge machine learning models.
As the field evolves, so too will this list. Your insights matter to us—so if you've experimented with a library that you think deserves a spot here, don't hesitate to mention it in the comments. We intend to update this guide regularly, incorporating tried-and-true tools that we and the community find invaluable for data science projects.
Take Action: Stay Updated and Engage with Us
Don't want to miss out on future updates and insights?
Remember to visit our blog for fresh perspectives on data science, machine learning, and technology. If you're grappling with a challenging data science issue or looking for tailored solutions, contact us at Sunscrapers. We're more than happy to help you navigate your data science journey.