
Maria Chojnowska
24 August 2023, 3 min read
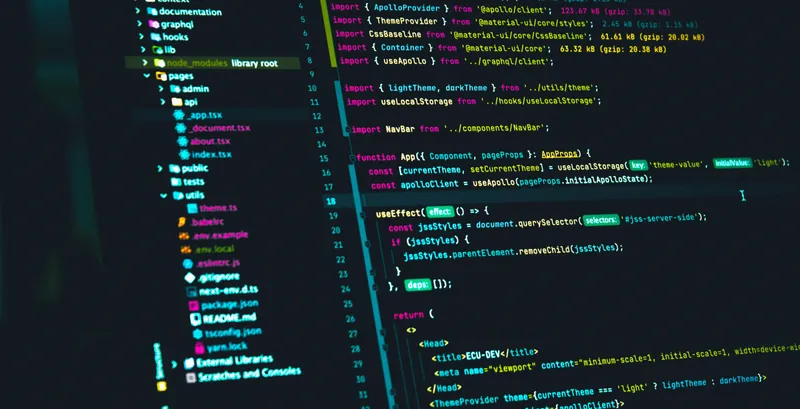
What's inside
- Matplotlib: The Heart of Python Visualization
- Installing and Importing Matplotlib
- Matplotlib Basics: Creating a Simple Line Plot
- Exploring Different Plot Types
- Customizing Your Plots
- Working with Multiple Plots
Matplotlib: The Heart of Python Visualization
Matplotlib is essentially a multi-platform data visualization library built on NumPy arrays, and designed to work with the broader SciPy stack. It was conceived by John Hunter in 2002, inspired by the MATLAB programming language, to enable interactive and easy creation of plots.
Installing and Importing Matplotlib
To install Matplotlib, we'll use pip, Python's package installer. In your terminal, type:
pip install matplotlib
To import Matplotlib, we generally import the `pyplot` submodule using the alias `plt`:
import matplotlib.pyplot as plt
Matplotlib Basics: Creating a Simple Line Plot
For the sake of demonstration, let's use a simple line plot. Let's assume we have time (in seconds) and the corresponding distance (in meters) travelled by an object.
# Importing required libraries
import matplotlib.pyplot as plt
# Create two lists, time (0 to 5 seconds) and distance (in meters)
time = [0, 1, 2, 3, 4, 5]
distance = [0, 1, 4, 9, 16, 25]
# Create a line plot
plt.plot(time, distance)
# Display the plot
plt.show()
Exploring Different Plot Types
Now, let's explore different types of plots that can be created using Matplotlib: 1. Histograms: These are useful for understanding the distribution of continuous numerical data.
import numpy as np
# Generating 1000 random values
random_data = np.random.randn(1000)
# Creating histogram
plt.hist(random_data, bins=20)
# Displaying the histogram
plt.show()
2. Scatter Plots: These are used to understand the relationship or correlation between two numerical variables.
# Generating 100 random values for x and y
x = np.random.rand(100)
y = np.random.rand(100)
# Creating scatter plot
plt.scatter(x, y)
# Displaying the scatter plot
plt.show()
3. Bar Plots: These are used to compare quantities of different categories.
# Creating a list of categories and their values
categories = ['A', 'B', 'C', 'D', 'E']
values = [7, 12, 6, 9, 14]
# Creating bar plot
plt.bar(categories, values)
# Displaying the bar plot
plt.show()
Customizing Your Plots
Visual aesthetics are a vital part of data visualization. Matplotlib allows us to control line styles, font properties, axes properties, etc. Let's customize the line plot we made earlier:
plt.plot(time, distance, color='purple', linestyle='--', linewidth=2)
# Adding title and labels
plt.title('Distance over Time')
plt.xlabel('Time (seconds)')
plt.ylabel('Distance (meters)')
# Adding a legend
plt.legend(['Object 1'])
# Display the plot
plt.show()
Working with Multiple Plots
In some scenarios, we might want to display multiple plots in one figure for comparison. Matplotlib provides the subplot()
function for this:
# First subplot
plt.subplot(1, 2, 1)
plt.plot(time, distance, 'r-')
plt.title('Plot 1: Line Plot')
# Second subplot
plt.subplot(1, 2, 2)
plt.scatter(time, distance, color='blue')
plt.title('Plot 2: Scatter Plot')
# Adjust distance between the two plots
plt.tight_layout()
# Show the plots
plt.show()
Remember, data visualization is not about creating flashy plots but about conveying information effectively. The best visualization method will depend on the data, its complexity, and the audience's needs.
Stay tuned for our next post, where we'll dive into advanced topics of Matplotlib, like 3D plotting, animation, and interactive plotting.
In the meantime, feel free to contact us if you need help with Python-related tasks, data visualization, machine learning, web development, or anything else. With our expertise, we can transform your ideas into working solutions.
Don't let Python's intricacies slow down your project. Contact us today for a discussion about your Python development needs.
Remember, the possibilities with Python and our professional services are limitless. Contact us.