
Kamil Niski
13 November 2023, 7 min read
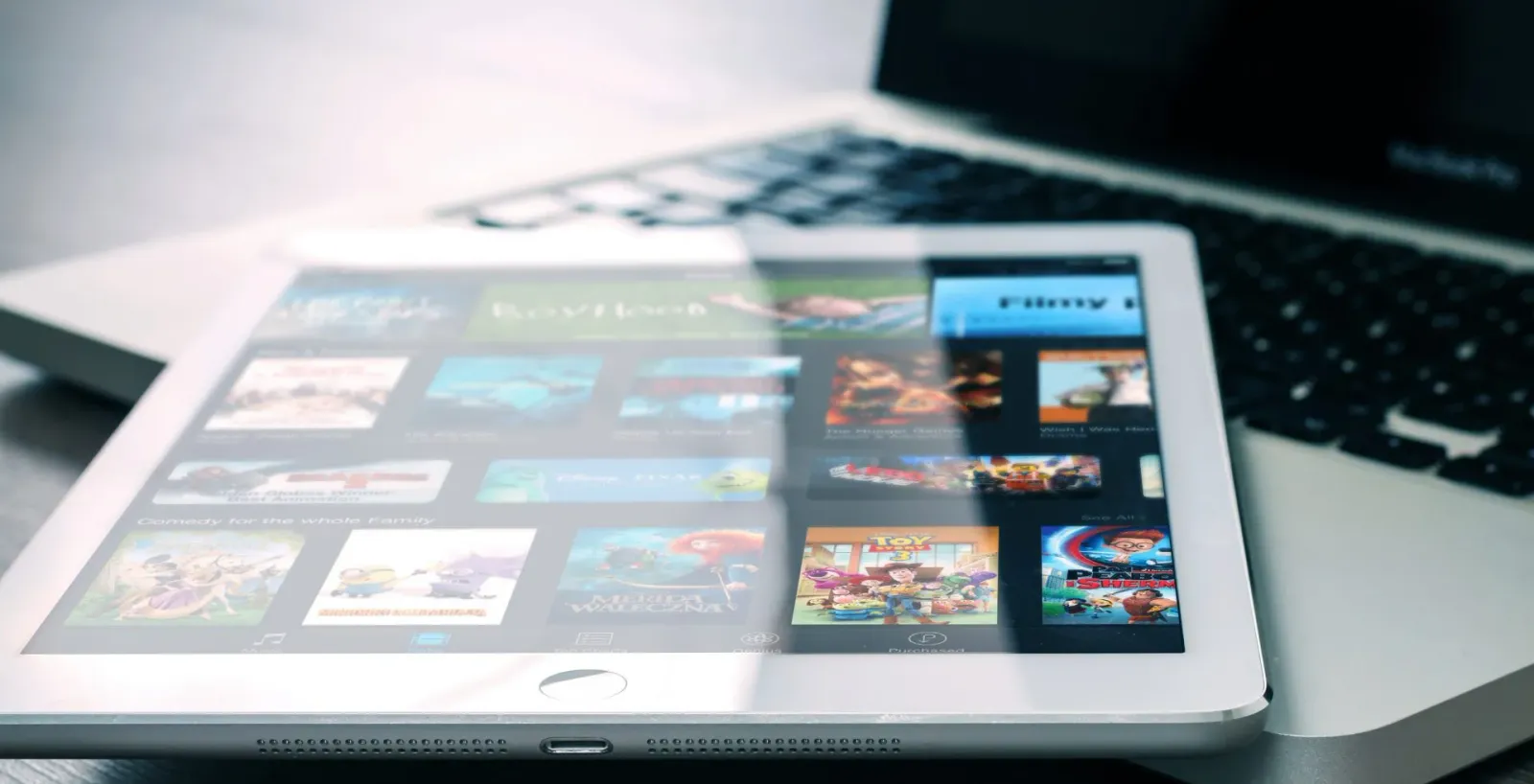
What's inside
- Recommendation Systems: Quick Primer
- From Data to Decisions: The Workflow of Recommendation Systems
- The Python Advantage in Building Recommendation Systems
- Advanced Insights for Seasoned Pythonistas
- Developing a Recommendation System: What To Consider
- Code Sample: A Basic Content-Based Recommender in Python
- In Conclusion
- Reach Out to Sunscrapers: Your Trusted Tech Partner
Recommendation systems are integral to our online experiences, seamlessly guiding our choices in movies, shopping, or content consumption. At Sunscrapers, our developers have found that delving into recommendation systems with Python leads to significant skill enhancement and innovative application development. Let's dive into the essentials.
Recommendation Systems: Quick Primer
Recommendation systems, or engines, are specialized information filters predicting a user's preferences toward an item, be it a movie, a product, or an article. The core types include:
- Simple Recommenders – this recommendation system offers generalized recommendations based on a product's popularity or rating score. The idea behind it is that, generally, products that are more popular and get higher ratings from users are more likely to be liked by the average audience.
- Content-based Recommenders – this recommendation system suggests similar items based on a particular item. The system uses metadata to provide these suggestions. For example, proposing a movie will use information such as the director, actors, genre, or description. This recommender system type assumes that if a user liked a particular item, they might also want a similar item – for example, a movie of the same genre or by the same director.
- Collaborative Filtering Engines – these systems are designed to predict the preference or rating a user would give to an item based on past preferences and ratings of other users. Contrary to content-based recommenders, collaborative filtering engines don't require item metadata.
From Data to Decisions: The Workflow of Recommendation Systems
Recommendation systems are pivotal in curating personalized user experiences across various digital platforms. This diagram illustrates the intricate process behind these systems, starting with raw data collection and culminating in curated suggestions for the end user. Through data processing and recommendation generation, the system refines vast amounts of data to offer pinpointed recommendations. Dive to understand the seamless workflow that powers your everyday content, shopping, and entertainment choices.
- Data Ingestion: Capturing and importing data from various sources for the recommendation system.
- Data Processing: Cleaning, transforming, and organizing raw data to extract meaningful patterns and insights.
- Recommendation Generation: Utilizing algorithms and models to predict and produce a list of items that users might find relevant.
- Simple Recommenders: Offering generalized suggestions based on an item's popularity or user ratings.
- Content-based Recommenders: Proposing items similar to ones the user has shown interest in based on shared attributes or characteristics.
- Collaborative Filtering Engines: Predicting a user's interests by analyzing preferences or behaviors of similar users.
- Post-Processing: Refining and optimizing the generated recommendations based on specific criteria or constraints.
- Delivery to End-User: Presenting the final recommendations to the user through an interface or platform.
- Feedback Loop: Gathering user reactions and responses to the recommendations, providing valuable insights for system improvement.
The Python Advantage in Building Recommendation Systems
Python is a popular interpreted language that, in combination with machine learning, has become one of the most common methods for building recommendation systems. Knowing Python is a huge advantage if you want to start a career in data science today.
Python's benefits in building recommendation systems are numerous. Here are some key reasons:
- Ease of Coding and Testing – since Python is such a productive language, it helps developers to write and test code easily. That, in turn, helps in dealing with sophisticated machine-learning algorithms. Not to mention that it's very flexible, and integrating different types of data or applying them to an existing operating system is relatively straightforward.
- Robust Libraries – a library is a collection of methods and functions allowing developers to perform many actions without writing code. Python offers many libraries that help developers implement machine learning in their projects.
- Vibrant Community – At Sunscrapers, we're part of the vibrant Python community, with developers passionate about machine learning and other innovative projects. Moreover, Python is an open-source language, and plenty of online material enables quick access to knowledge relevant to machine learning. That makes Python an excellent pick for any project that focuses on building a recommendation system. Since you're building a machine learning-based system, expect it to take a lot of time to develop and fine-tune. But it's also worth to enjoy the learning process!
Advanced Insights for Seasoned Pythonistas
For the adept Python developer, the realm of recommendation systems transcends basic algorithms. The intersection of deep learning with recommendation engines, through techniques like Neural Collaborative Filtering, offers profound precision. Moreover, embracing hybrid models captures the collaborative and content-based worlds best. Scalability challenges are met with platforms like Spark, and ethical coding becomes paramount to avoid biases in recommendations. Additionally, specialized toolkits like LightFM and Surprise cater to sophisticated experimentation, marking the field expansive and thrilling.
Developing a Recommendation System: What To Consider
For those looking to venture into building recommendation systems using Python, here are a few considerations:
- Choice of Recommender Type - Your selection will hinge on your data and the problem statement. Collaborative filtering may be ideal if you rely on user interactions without much metadata. Content-based recommendations might be the way forward if you have rich item metadata.
- Data Source - Your recommendation engine's effectiveness is only as good as the data feeding it. Popular datasets like the MovieLens dataset are excellent for initial experiments, but real-world applications will need robust and clean datasets for training.
- Performance Metrics - Track the efficiency of your recommendation system using metrics like RMSE (Root Mean Squared Error), precision@k, and recall@k, adjusting and tuning your model accordingly.
Code Sample: A Basic Content-Based Recommender in Python
Here's a simple content-based recommender using Python to give a practical touch. This method leverages item metadata, such as descriptions or features, to recommend similar items. In this example, we use the TfidfVectorizer from scikit-learn to transform movie descriptions into numerical data and then compute the similarity scores between movies.
import pandas as pd
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.metrics.pairwise import linear_kernel
# Sample data
data = {'movie': ['Movie1', 'Movie2', 'Movie3'],
'description': ['Action thriller', 'Romantic comedy', 'Action romance']}
df = pd.DataFrame(data)
# Compute the TF-IDF matrix
tfidf = TfidfVectorizer(stop_words='english')
df['description'] = df['description'].fillna('')
tfidf_matrix = tfidf.fit_transform(df['description'])
# Compute cosine similarity
cosine_sim = linear_kernel(tfidf_matrix, tfidf_matrix)
# Get movie recommendations based on cosine similarity
def get_recommendations(title, cosine_sim=cosine_sim):
idx = df.index[df['movie'] == title].tolist()[0]
sim_scores = list(enumerate(cosine_sim[idx]))
sim_scores = sorted(sim_scores, key=lambda x: x[1], reverse=True)
sim_scores = sim_scores[1:3]
movie_indices = [i[0] for i in sim_scores]
return df['movie'].iloc[movie_indices]
print(get_recommendations('Movie1'))
For those looking to delve deeper, let's explore the Surprise library - a Python scikit for building and analyzing recommendation systems. In this example, we'll use Singular Value Decomposition (SVD), a popular matrix factorization method, to predict user ratings.
from surprise import SVD, Dataset, Reader, accuracy
from surprise.model_selection import train_test_split
# Create a sample dataset
ratings = {
'user': ['A', 'A', 'B', 'B', 'C', 'C'],
'item': ['Item1', 'Item2', 'Item1', 'Item3', 'Item2', 'Item3'],
'rating': [1, 2, 2, 3, 3, 1]
}
df = pd.DataFrame(ratings)
# Define a reader and the scale of ratings
reader = Reader(rating_scale=(1, 3))
# Load the dataset from the DataFrame
data = Dataset.load_from_df(df, reader)
# Split data into training and test set
trainset, testset = train_test_split(data, test_size=0.2, random_state=42)
# Train an SVD model
model = SVD()
model.fit(trainset)
# Predict ratings for the test set
predictions = model.test(testset)
# Calculate RMSE for the predictions
rmse = accuracy.rmse(predictions)
print(f"Test Set RMSE: {rmse:.3f}")
In Conclusion
From our extensive work at Sunscrapers, we've seen firsthand how well-constructed recommendation systems can profoundly enhance user experiences. Python offers a robust foundation for developers to craft these systems with precision and efficiency. As you embark on this journey, remember that continuous learning, experimentation, and fine-tuning are key. Enjoy the process!
Reach Out to Sunscrapers: Your Trusted Tech Partner
As you embark on the thrilling journey of building recommendation systems using Python, remember that you're not alone. Sunscrapers, with its deep expertise in Python and machine learning, is your ideal tech partner.
Whether you're seeking guidance, collaboration, or a comprehensive solution, our team at Sunscrapers can amplify your efforts and enhance the results. Our extensive experience and proactive approach ensure that your project receives the best possible technological inputs.
Don't let challenges hinder your progress. Connect with Sunscrapers today and leverage the power of Python and machine learning for superior recommendation systems.
Contact Sunscrapers now, and let’s turn your ideas into groundbreaking solutions!