
Maria Chojnowska
14 September 2023, 5 min read
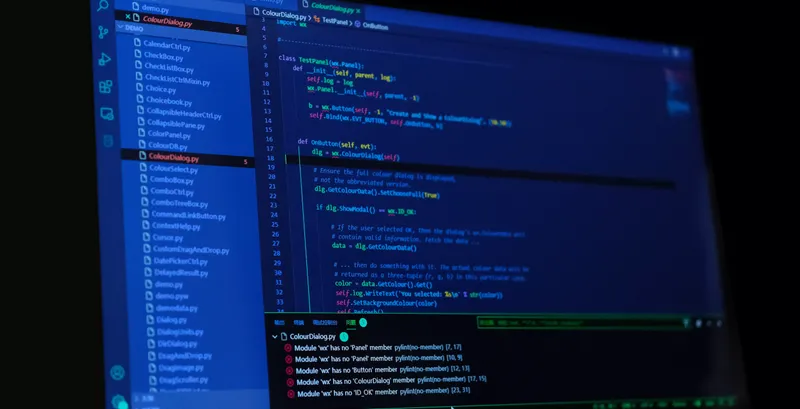
What's inside
- Python Code Profiling: The Key to Identifying Performance Bottlenecks
- Optimizing Memory Management in Python: Generators and Garbage Collection
- How to Optimize Python Loops: Unrolling and Vectorization
- Python Concurrency: Threading and Asynchronous Programming for Performance
- Leveraging GPU Acceleration and Machine Learning for Python Performance Optimization
- Wrapping Up: Next Steps for Python Performance Optimization
- TL;DR: Quick Takeaways for Python Performance and Code Quality Optimization
For the seasoned Python developer, the notion of performance optimization is a familiar landscape—fraught with challenges yet abundant with opportunities for innovation. While churning out Python code that 'works' is easy, crafting code that performs optimally is an art. This article is not for beginners but is tailored for professionals interested in taking their Python optimization techniques to the next level.
If you're pondering questions like:
- How do I optimize Python code for performance?
- How do I improve the quality of my Python code?
- What are the best tools for optimizing Python code?
Then read on. We’ll delve deep into the advanced techniques for Python optimization techniques and discuss how our optimization services can help you take these strategies to scale.
Python Code Profiling: The Key to Identifying Performance Bottlenecks
Before you embark on the optimization journey, identifying your bottlenecks is crucial. With Python, you're in luck. Python provides a rich set of libraries specifically designed for profiling.
cProfile: A Standard for Performance Profiling
For time-based profiling, ‘cProfile’
is a go-to. It's a built-in Python library and a powerful tool that provides more detailed insights than the ‘time’ module.
import cProfile
def my_function():
# Your complex code here
pass
cProfile.run('my_function()')
Py-Spy: Sampling Profiler for Great Insights
For real-time profiling without modifying the code, Py-Spy is incredibly useful, especially for running Python programs. With Py-Spy, you can analyze CPU utilization and other performance metrics easily.
pip install py-spy
py-spy -- python your_program.py
Optimizing Memory Management in Python: Generators and Garbage Collection
Generators: Memory Efficiency in Iterables
Using generators can significantly reduce memory consumption. Generators are iterators that yield items on the fly and do not store the entire iterable in memory.
def my_large_range(n):
i = 0
while i < n:
yield i
i += 1
Garbage Collection: Manual Control
Taking manual control of Python's garbage collector can be beneficial for short-lived objects that you know will go out of scope soon.
import gc
gc.disable()
# your high-performance code
gc.enable()
How to Optimize Python Loops: Unrolling and Vectorization
While Python’s syntactical simplicity makes it a top choice for developers, its interpreted nature can make loops painfully slow. Loop unrolling and vectorization are two approaches to improve loop performance drastically.
Loop Unrolling
This involves manually expanding the iterations in a loop, minimizing the overhead of loop control code.
for i in range(0, len(my_list), 4):
process(my_list[i])
process(my_list[i+1])
process(my_list[i+2])
process(my_list[i+3])
Vectorization with NumPy
NumPy's array operations are implemented in C, providing a speedup over traditional Python loops.
import numpy as np
array1 = np.array([1, 2, 3, 4])
array2 = np.array([5, 6, 7, 8])
sum_array = np.add(array1, array2)
Python Concurrency: Threading and Asynchronous Programming for Performance
Threading with ‘concurrent.futures’
For I/O-bound problems, Python’s ‘concurrent.futures’ module offers an elegant way to create a thread pool and parallelize tasks.
from concurrent.futures import ThreadPoolExecutor
def fetch_data(x):
# Simulate some I/O-bound operation
return x * x
with ThreadPoolExecutor() as executor:
results = list(executor.map(fetch_data, range(10)))
Asynchronous Programming with ‘asyncio’
For high-level asynchronous I/O handling, ‘asyncio’ is a powerful library. It's particularly beneficial for scalable I/O-bound tasks.
import asyncio
async def my_coroutine(n):
await asyncio.sleep(1)
print(f"Coroutine {n}")
async def main():
await asyncio.gather(
my_coroutine(1),
my_coroutine(2),
my_coroutine(3)
)
asyncio.run(main())
Leveraging GPU Acceleration and Machine Learning for Python Performance Optimization
GPU Acceleration with CuPy
For numerical computations, CuPy leverages the GPU’s computational capabilities, offering a significant performance boost compared to CPU-based solutions.
import cupy as cp
x = cp.array([1, 2, 3])
y = cp.array([4, 5, 6])
z = x + y
Machine Learning with TensorFlow
TensorFlow provides comprehensive support for various optimization algorithms that can fine-tune your machine learning models for performance.
import tensorflow as tf
model = tf.keras.Sequential([
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam', loss='categorical_crossentropy')
Wrapping Up: Next Steps for Python Performance Optimization
Performance optimization in Python is a rich and rewarding field, laden with opportunities for innovation. Profiling, memory management, loop optimization, parallelism, and leveraging specialized hardware are not just techniques but necessities for anyone serious about Python performance.
Are you looking to leverage these advanced techniques in your projects at scale? Our optimization services are specially designed to help businesses improve their Python codebase for performance, maintainability, and scalability.
Your Next Steps for Advanced Python Code Optimization
If you’re interested in our optimization services, reach out to us for an initial consultation.
- Tailored Solutions:
We offer customized solutions that fit your specific needs.
- Long-Term Support:
Our team provides ongoing support to ensure your code stays optimized and up-to-date.
Elevate your Python game to the next level with our services. We're here to guide you through the labyrinth of optimization toward a future of blazingly fast, highly efficient Python code.
TL;DR: Quick Takeaways for Python Performance and Code Quality Optimization
If you're looking for straightforward answers to some key questions about Python code optimization, here's a summary:
- How do I optimize Python code for performance?
Use profiling tools like ‘cProfile’ and ‘Py-Spy’, manage memory with generators and garbage collection, optimize loops through unrolling and vectorization, and employ concurrency and hardware acceleration.
- How do I improve the quality of my Python code?
Adopt good profiling and memory management practices, streamline your code structure, and utilize concurrent and asynchronous programming for scalability and maintainability.
- What are the tools for optimizing Python code?
For profiling, use ‘cProfile’
and ‘Py-Spy’
. For memory management, utilize generators and the ‘gc’
module. Optimize loops with ‘NumPy’
. For concurrency, rely on ‘concurrent.futures’
and ‘asyncio’
. Use ‘CuPy’
for GPU acceleration and ‘TensorFlow’
for machine learning optimization.
Consider our optimization services for more details and how to leverage these techniques at scale. Reach out for an initial consultation to elevate your Python game to the next level.