
Patryk Młynarek
10 January 2024, 9 min read
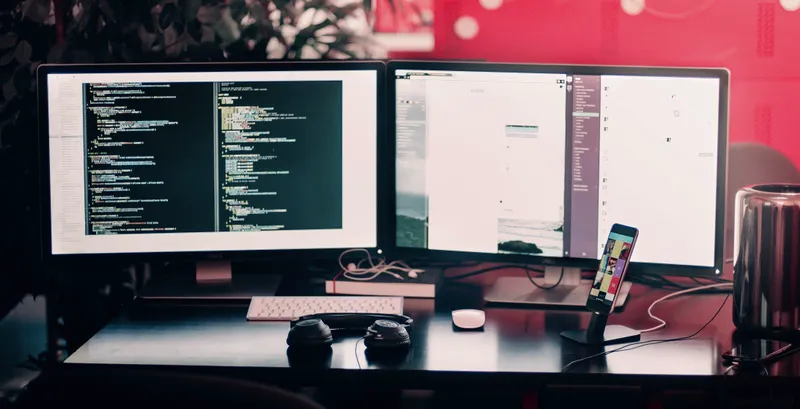
What's inside
- Tech Overview
- The Power Behind the Combination
- Getting Started: Installing the Powerhouse
- Ensuring Security: CORS
- Showcase Project
- Key Features
- Conclusion
In the ever-evolving world of web development, the quest is always to create apps that are not just fast and interactive but also visually appealing. This is where the powerful combination of FastAPI, HTMX, Jinja2, DaisyUI, and Tailwind CSS steps in.
This article explores why this mix is fantastic, exploring what each part does best. From setting up and showing code examples to keeping things secure and a real-life project on GitHub, get ready for a journey that mixes speed, interaction, and style to boost your web development.
Explore the full implementation and details in our dedicated repository for an in-depth look into the article's concepts and examples.
Tech Overview
Let's quickly navigate FastAPI, HTMX, Jinja2, DaisyUI, and Tailwind CSS.
Explore their unique capabilities, from efficient backend processes to dynamic frontend interactions.
FastAPI
FastAPI is a high-performance Python web framework designed for rapid web development, combining Python-type hints with automatic OpenAPI generation.
HTMX
HTMX is a lightweight JavaScript library facilitating seamless server-client communication, simplifying real-time updates, and enhancing user interactions.
Jinja2
Jinja2 is a Python templating engine that enables the generation of dynamic content in web applications, providing an expressive syntax for HTML templates.
Tailwind CSS and DaisyUI
Tailwind CSS, known for its utility-first approach, lays the foundation for versatile and flexible styling in web development. DaisyUI, positioned as a plugin and component library built on top of Tailwind CSS, introduces a groundbreaking paradigm in UI design.
This dynamic relationship positions DaisyUI not just as a companion but as a crucial extension, providing an extensive array of ready-made elements
comparable to the richness of Bootstrap and operating harmoniously alongside Tailwind CSS. This innovative synergy redefines UI development and positions DaisyUI as a compelling alternative to traditional frameworks.
It seamlessly balances Tailwind CSS's super flexibility with DaisyUI's efficiency in delivering polished, ready-to-use components. It offers a powerful toolkit for visually appealing and highly functional interfaces with remarkable ease.
The Power Behind the Combination
Modern World of Development
Let's dive into the modern development world. These technologies—FastAPI, HTMX, Jinja2, DaisyUI, and Tailwind CSS—are relatively new in web development but bring in the latest standards and quality, often missing in older tools. Despite being recent, it's crucial to highlight their stability. These tools are proven and reliable for real-world applications, adept at incorporating the best from other technologies.
Rapid Development
Moving ahead, we step into rapid development. Each technology is crafted for seamless integration. FastAPI handles automatic documentation, and Tailwind CSS + DaisyUI delivers pre-built components without needing JavaScript, thanks to HTMX. By combining Jinja2 and FastAPI, we unlock the benefits of server-side rendering streamlining development, proving particularly advantageous for efficient prototyping.
Simplicity and Ease of Maintenance
The third strength lies in a straightforward tech stack that simplifies maintenance. Having the visual (Frontend) and functional (Backend) aspects in one place makes long-term project upkeep easy, potentially lowering costs.
Flexibility and Customization
The strength of the stack lies in its exceptional flexibility and ease of customization. Tailwind CSS, celebrated for its adaptability, allows developers to adjust the visual aspects of the application intricately. This flexibility seamlessly extends to DaisyUI's components, complementing Tailwind CSS's utility-first approach.
Beyond the UI, the frontend layer with HTMX and its lightweight structure provides flexibility in building dynamic and interactive user interfaces. It decreases both architecture and code complexity as there is no need for dedicated APIs or state management. It moves the balance towards the backend side.
The main idea behind HTMX requires the backend to respond with HTML-rendered partials. Jinja2, as the templating engine, gives everything that is needed, even more thanks to its extensibility.
The backend built with FastAPI gives high performance and plenty of space for customization or architecture design.
Speed and Efficiency
It's built for speed. FastAPI + HTMX ensures rapid server-side requests and consumption. Jinja2, being efficient and seamlessly integrated with FastAPI, enhances server-side rendering.
Easy Setup and Deployment
Setting up and initializing the project is a breeze, especially when deploying with Docker. This simplicity streamlines the development process from start to finish.
Visual Appeal
The visual elements of the tech stack shine. Tailwind CSS + DaisyUI offers a modern and visually appealing design. HTMX's lightweight nature complements Tailwind CSS + DaisyUI, allowing the integration of interactive elements without sacrificing performance or introducing unnecessary complexity.
Getting Started: Installing the Powerhouse
Get ready to dive into the dynamic world! Follow these steps to install and set up this powerful combination for your web development needs. Let's get started!
Installing FastAPI
pip install fastapi
For more information, refer to the FastAPI documentation.
Installing HTMX
<!-- Add this script tag to your HTML file -->
<script src="https://unpkg.com/htmx.org@1.9.9/dist/htmx.min.js"></script>
For more information, refer to the HTMX documentation. The presented solution is not recommended for production usage. Follow official documentation for more information.
Installing Jinja2
pip install jinja2
For more information, refer to the FastAPI Templates docs and Jinja2 docs.
Installing Tailwind CSS and DaisyUI
<!-- Add this to your HTML file -->
<link href="https://cdn.jsdelivr.net/npm/daisyui@4.4.23/dist/full.min.css" rel="stylesheet" type="text/css" />
<script src="https://cdn.tailwindcss.com"></script>
For detailed documentation, refer to Tailwind CSS and DaisyUI. CDN files are not recommended for production. Make sure to follow official docs.
Docker Approach
Using Docker simplifies the setup of your development environment, ensuring consistency across different systems. The provided Dockerfile encapsulates dependencies, making sharing and deploying your application easy.
Dockerfile Example:
# Use an official Python runtime as a parent image
FROM python:3.11.7-slim
# Install Node.js
RUN apt update && apt install -y nodejs npm
# Install Python requirements
ADD requirements.txt requirements.txt
RUN pip install requirements.txt
# Copy project
ADD /app /src/app
ADD /tailwindcss/ /src
# Set workdir
WORKDIR /src
# Install Tailwind CSS and DaisyUI
# Make sure to follow official docs to fulfill installation
RUN npm install tailwindcss
RUN npm install daisyui
# Command to run when image started
CMD uvicorn app.main:app --host 0.0.0.0 --port 8000 --reload
Note: Adjustments to this Dockerfile may be needed based on your project.
Ensuring Security: CORS
In considering the security of our application and fortifying it against potential vulnerabilities, let's delve into CORS. As we navigate the complexities of modern web security, it's important to understand and implement Cross-Origin Resource Sharing (CORS) to safeguard our application from potential cross-domain attacks. In the subsequent part, we will also cover CSRF Token protection, further enhancing our application's security measures against common web threats. Stay tuned for a comprehensive approach to securing your web applications.
CORS (Cross-Origin Resource Sharing)
Cross-Origin Resource Sharing is a crucial security feature implemented by web browsers to regulate resource access across different domains. As our application expands and handles sensitive data, the introduction of CORS becomes paramount. However, it's important to note that the application will function properly without this configuration. Here's an example implementation using the starlette.middleware.cors
middleware:
from fastapi import FastAPI
from starlette.middleware.cors import CORSMiddleware
app = FastAPI()
# Configure CORS
app.add_middleware(
CORSMiddleware,
# Update with specific origins in production
allow_origins=["localhost"],
allow_methods=["GET", "POST"],
)
In this example, allow_origins
can be customized to specific origins in production, ensuring a controlled environment for your application.
Note: Always analyze your project needs concerning security. Additional security mechanisms might be required depending on the nature of your application.
Showcase Project
Dive into the practical application of FastAPI, HTMX, DaisyUI, and more by exploring our showcase project. This repository is not just code; it's a starting point for your innovative creations.
Download the repository, experiment with its features, and adapt it to your needs. Our showcase provides a real-world context to understand the power and flexibility of this tech stack.
Get started now and see what you can build! Download the repository.
Project Structure In Short
.
├── Makefile
├── README.md
├── app
│ ├── core
│ ├── db.py
│ ├── main.py
│ ├── routes.py
│ ├── static
│ ├── templates
│ ├── tests
│ ├── utils.py
│ └── views
├── docker
├── docker-compose.yml
├── example.env
├── requirements
└── tailwindcss
Highlights
- Makefile: Streamline project management with predefined commands for easy execution.
- Dockerfile: A production-ready Dockerfile, ensuring seamless deployment with minimal adjustments.
Technologies Utilized
- FastAPI: Powering the backend with speed, simplicity, and asynchronous capabilities.
- HTMX: Enabling dynamic updates with minimal JavaScript and seamless server-client communication.
- Jinja2: Facilitating server-side rendering for dynamic content generation.
- Tailwind CSS + DaisyUI: Crafting visually appealing and highly interactive user interfaces with ready-to-use components.
- Docker: Ensuring consistency in development and deployment environments.
Key Features
- Dynamic Static Files Generation: Implemented in docker-compose.yml for HTMX, Tailwind CSS + DaisyUI, and FastAPI application.
- Makefile Convenience: Predefined commands for easy project management.
- Tested with Pytest: Robust testing using Pytest to maintain code quality.
- Configurable Application: Easily customizable via configuration file.
- TODO App: A practical implementation of a TODO application for real-world demonstration.
- Pre-commit Hook: Enforce code quality and standards with a pre-commit hook.
- Lazy Loading of Data: Efficiently implemented lazy loading of the tasks view with a spinner indicating data loading.
- Out of Band Updates: Utilizing HTMX for updates "Out of Band" for a seamless user experience.
- Organized Templates: Templates organized in subfolders for better maintenance.
Consider this showcase project your sandbox — tweak, fine-tune, and adjust every aspect to fit your coding preferences. Dive into the codebase and make it yours, tailoring each element to align with your project's specific needs and your coding style.
Conclusion
As we've explored, the powerful combination of FastAPI, HTMX, Jinja2, Tailwind CSS, DaisyUI, and Docker offers a unique blend of speed, efficiency, and design flexibility that sets new standards in modern web development.
Embrace this technological synergy to create web experiences that are visually stunning and highly interactive but also robust and scalable.
I encourage you to download the showcase project, tailor it to your needs, and share your innovations and experiences. Your contributions can inspire others and help evolve the ecosystem of web development technologies. So go ahead, code confidently, and let's build the future of the web together!
Download the repository and start crafting your next masterpiece today.