
Maria Chojnowska
8 September 2023, 12 min read
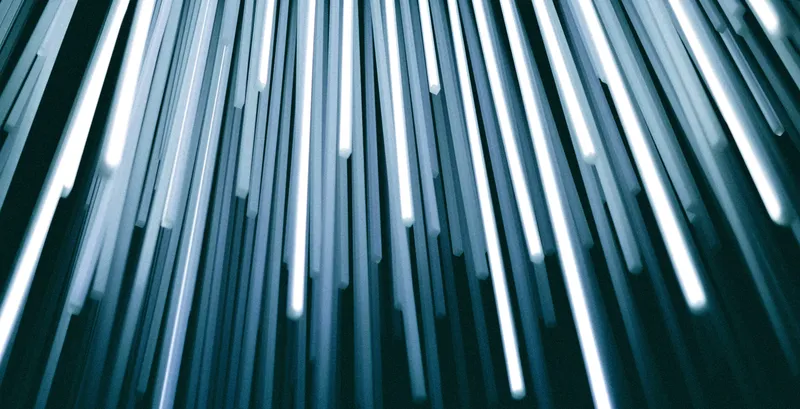
What's inside
- Introduction to pdb
- Features and Capabilities of pdb
- Setting up pdb in Your Development Environment
- Detailed Guide to Using pdb Commands
- Debugging Python Applications with pdb: Real-World Case Studies
- Common Issues and How to Solve Them with pdb
- Augmenting pdb with Third-Party Tools
- Conclusion: Is pdb a Good Debugger?
- Unravel the Power of Python with the Best!
In the world of programming, the unfortunate truth is that bugs are inevitable. They can be as minor as syntax errors or as complex as logical flaws.
And when it comes to Python — a language renowned for its readability and efficiency — debugging takes on unique challenges and opportunities.
Debugging is the medical diagnosis in the software world, systematically treating the issues plaguing your code to ensure smooth operation.
In this comprehensive guide to debugging Python apps, we'll explore the ins and outs of using pdb effectively. So whether you're a Python novice or a seasoned developer, read on to elevate your debugging game.
Introduction to pdb
Enter pdb, Python's built-in interactive source debugger. The acronym 'pdb' stands for Python DeBugger, embodying its core function. Conceived as part of Python's standard library, pdb equips developers with an accessible and powerful tool to spot bugs effectively.
Unlike the stereotypical picture of complex and clunky debugging tools, pdb is elegance personified. It provides an interactive debugging environment, allowing you to execute commands step by step, inspect variables, and modify their values - all through a simple yet effective command-line interface.
Through pdb, debugging transforms into a journey of understanding and learning. It enables us to delve into our code's depths, exploring its behavior, dissecting the bugs, and enlightening us about the pathways our program traverses.
As we go along with this article, remember: pdb isn't just a tool.
It's a compass, guiding us through the uncharted territories of our Python code, revealing the hidden bugs, and helping us navigate towards clean, efficient code.
Features and Capabilities of pdb
The strength of pdb lies in its rich array of features and capabilities, facilitating an efficient debugging experience.
One of the key features of pdb is the creation of breakpoints. By inserting pdb.set_trace()
at any point in
your code, you can pause the execution of the program, making it a potent tool to narrow down the source of bugs.
Upon reaching the breakpoint, pdb provides an interactive session, allowing you to inspect the variables, execute
commands, and modify the values if necessary.
Next is the ability to step through the code. Pdb provides commands like next, step, and continue, which give granular control over the program execution. Whether it's about stepping over, stepping into a function, or resuming the program until the next breakpoint, pdb has got you covered.
What if you want to inspect the values of certain variables when a condition is met? Pdb caters to this through watchpoints. This advanced feature lets you define conditions that, when met, trigger an automatic breakpoint, making it easier to catch elusive bugs.
import pdb
def calculate_sum_of_squares(n):
total = 0
for i in range(n+1):
if i == 5:
pdb.set_trace()
total += i * i
return total
print(calculate_sum_of_squares(10))
Pdb also shines with its post-mortem debugging capabilities. This feature allows pdb to handle uncaught exceptions and enter post-mortem debugging, proving to be a boon in scenarios where you didn't set a breakpoint but want to investigate an exception.
Lastly, pdb allows you to save and load breakpoints and commands across debugging sessions, adding a touch of persistence to your debugging process.
import pdb
def risky_division():
try:
result = 10 / 0
except ZeroDivisionError as e:
pdb.post_mortem()
print("An error occurred:", e)
risky_division()
Setting up pdb in Your Development Environment
Getting pdb up and running is a breeze, given that it is a part of Python's standard library. It doesn't require any complex installations or configurations. If you have Python installed in your development environment, you already have pdb. The only task left is to incorporate it into your code.
To employ pdb in your program, simply import it at the beginning of your script with import pdb. You can set a
breakpoint at any suspected part of your code by calling pdb.set_trace()
. This statement tells Python to pause
execution at that point and provide an interactive pdb debugging session.
Here's a sample of what a pdb setup might look like in a simple Python script:
import pdb
def buggy_function(n):
result = 0
for i in range(n):
pdb.set_trace()
result += i / (i - 4)
return result
print(buggy_function(10))
In this example, when buggy_function(10)
is executed, the program will halt at the line with **pdb.set_trace()
**, offering you an interactive pdb session. From this point, you can start inspecting variables, executing statements,
and stepping through the code.
Remember that pdb.set_trace()
merely sets a pause point, not a permanent stop. You can decide how the code
execution proceeds from this point using pdb commands. This capability provides unparalleled control over your debugging
process, enhancing your code diagnosis effectiveness.
import pdb
def find_factorial(n):
pdb.set_trace()
if n == 1:
return 1
else:
return n * find_factorial(n-1)
print(find_factorial(5))
Detailed Guide to Using pdb Commands
Pdb's power lies in its diverse set of commands. Each command serves a specific purpose, facilitating various aspects of the debugging process. Let's embark on a comprehensive tour of pdb's command toolkit.
Navigation Commands
Whether you're in a dense forest or debugging complex code, knowing how to navigate is crucial. In this section, we'll cover the fundamental commands that allow you to step through your Python code. One line or function at a time.
n(ext)
: Execute the next line and stop execution.s(tep)
: Step into a function, method, or class.r(eturn)
: Continue execution until the current function's return is reached.c(ont(inue))
: Continue execution until a breakpoint is encountered.j(ump) <lineno>
: Jump to a specified line in the current file.
Inspection Commands
Once you've navigated to a point of interest in your code, you'll want to take a closer look. Inspection commands in pdb let you examine the state of variables, arguments, and even the call stack. Here's your magnifying glass into the world of Python debugging.
a(rgs)
: Print the argument list of the current function.p <expression
>: Evaluate the expression and print its value.pp <expression>
: Pretty-print the value of the expression.l(ist)
: List the source code around the current line.w(here)
: Print a stack trace, with the most recent call at the bottom.
Breakpoint Commands
Setting breakpoints is like marking waypoints on your debugging journey. It helps you pause execution where you suspect things might be going awry. Learn how to effectively set, clear, and manage breakpoints in this section.
b(reak) <lineno>
: Set a breakpoint at a specified line.b(reak) <filename:lineno>
: Set a breakpoint in another file.b(reak) <function>
: Set a breakpoint at the first executable line of a function.tbreak <lineno>
: Set a temporary breakpoint, which is removed automatically once hit.cl(ear) <breakpointno>
: Clear the specified breakpoint.
Post-mortem and Execution Commands
Sometimes, the debugging process necessitates a 'reboot' or even a complete exit. In this section, we'll cover the commands that help you control the overall flow of your pdb session, from restarting the debugging process to quitting it entirely.
run <args>
: Restart the debugged python program.q(uit)
: Quit pdb abruptly.
Note that the parentheses in the above commands denote the shorthand form. For instance, you can use n instead of next.
For inspecting the program's state, pdb offers args, p, pp, list, and where. args prints the argument list of the current function, p and pp print the value of an expression (the latter in a pretty format), list displays the source code around the current line, and where prints a stack trace of the execution.
Pdb also provides comprehensive control over breakpoints. With break, you can set a breakpoint at a specific line or function, and clear allows you to remove a breakpoint. The tbreak command sets a temporary breakpoint that automatically gets cleared once hit.
Lastly, pdb offers run and quit to control the debugging session. Run restarts the debugged python program, and aborts the pdb session abruptly.
By mastering these commands, you equip yourself with a potent toolkit to navigate the intricate maze of your Python code, unveiling the bugs lurking in its corridors, and ultimately, enhancing the health and efficiency of your program.
Debugging Python Applications with pdb: Real-World Case Studies
While pdb shines in debugging simple Python scripts, its true power emerges in more complex Python applications. Let's consider a more intricate case.
Imagine you're building a web application using Django. In one of your views, you encounter a bug causing unexpected behavior, but you can't determine the root cause. Here's where pdb becomes invaluable.
First, locate the view where the bug appears. Just before the point where you suspect the bug, insert import pdb; *
*pdb.set_trace()
**. Now, when you run your server and navigate to the particular view in your web browser, the server
will halt at the breakpoint, providing an interactive pdb session in your terminal.
# views.py in Django
from django.http import HttpResponse
def my_view(request):
my_variable = "Hello World"
import pdb; pdb.set_trace()
return HttpResponse(my_variable)
In this session, you have full access to the request and response objects, the database, and any variables or functions defined at that point in your view. You can print variables, modify them, call functions, and even perform database queries - all live in your terminal.
This detailed inspection ability can help you diagnose even the most elusive bugs. You can step into function calls, check variable values at various points, and execute code snippets to test your hypotheses about the bug's source. Once you've determined the root cause, you can modify your code to resolve it, ensuring your Django application runs smoothly.
In both case studies, pdb proved to be a powerful ally, shedding light on hidden bugs and providing valuable insights into the code's behavior. But debugging isn't just about identifying bugs; it's also about avoiding common pitfalls and adhering to best practices.
Common Issues and How to Solve Them with pdb
As powerful as pdb is, you may still encounter some issues during debugging. One common problem is forgetting to
remove pdb.set_trace()
after debugging, which might halt your program's execution unexpectedly in production.
To avoid this issue, always ensure to remove or comment out your breakpoints once you've resolved the bug. A good practice is to search for "pdb" in your code before committing or deploying to catch any lingering breakpoints.
Another common issue is dealing with multithreaded applications. Pdb doesn't handle multithreading well out of the
box; breakpoints in one thread may interrupt all threads. To work around this, you can use pdb.Pdb()
to create a
pdb object and set_trace
on it, isolating the breakpoint to the current thread.
import pdb
import threading
def print_numbers():
for i in range(10):
print(i)
if i == 5:
my_pdb = pdb.Pdb()
my_pdb.set_trace()
thread = threading.Thread(target=print_numbers)
thread.start()
Finally, pdb's command-line interface might not be user-friendly for beginners. However, various tools build on pdb to provide a more intuitive and feature-rich debugging experience. Tools like ipdb and pdb++ enhance pdb with syntax highlighting, tab completion, and improved navigation, making it more accessible for beginners.
Augmenting pdb with Third-Party Tools
Despite pdb's efficacy, it is, in essence, a minimalistic debugger, intended to provide only the necessary features for debugging Python code. For more complex tasks or a more user-friendly debugging experience, several third-party tools enhance pdb's functionality.
ipdb
IPython, a powerful interactive shell for Python, introduces ipdb, a debugger with the same interface as pdb but with added IPython features. It offers syntax highlighting, tab completion, improved help messages, and the ability to debug IPython-specific syntax, providing a more comfortable and efficient debugging experience.
You can install ipdb using pip:
pip install ipdb
To use ipdb, replace import pdb; pdb.set_trace()
in your code with import ipdb; ipdb.set_trace()
.
pdb++
Pdb++ is another pdb enhancement providing additional features and improvements over the base pdb. It offers syntax highlighting, sticky mode for better code navigation, several new commands for inspecting objects, and smart command parsing.
Install pdb++ with pip:
pip install pdbpp
To use pdb++, you don't need to change your code. Once installed, pdb++ will automatically be used when you invoke
import pdb; pdb.set_trace()
.
Conclusion: Is pdb a Good Debugger?
As a built-in Python tool, pdb comes with several advantages. It's available out of the box with Python, ensuring you don't need to worry about setup or compatibility issues. Its commands provide granular control over code execution, allowing for precise debugging. It's also extendable with third-party tools for enhanced functionality.
In summary, pdb is a reliable, versatile, and powerful debugger for Python. It can tackle anything from simple scripts to complex applications. It might not be the most glamorous tool, but it's a steadfast workhorse that, once mastered, can considerably boost your debugging efficiency and code quality.
So, is pdb a good debugger? Unequivocally, yes. As a Python developer, you owe it to yourself to understand and utilize pdb, your secret weapon in the ceaseless battle against bugs.
Happy Debugging!
Unravel the Power of Python with the Best!
Facing challenges with your Python projects? Whether it's sophisticated web apps or intricate data analytics, our team boasts the top Pythonistas in the field. Don't settle for average; let the experts guide you to success!
Reach out to us and experience unparalleled Python expertise. Let's transform your ideas into powerful solutions together!
Because when it comes to Python, we don't just code – we craft masterpieces.